How to Limit Characters on a Marketing Cloud Account Engagement (Pardot) Form Textarea
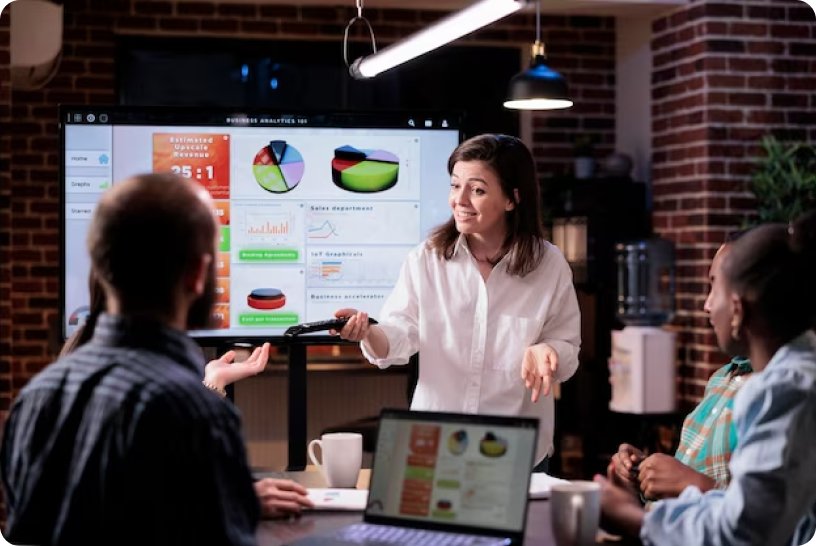
How to Limit Characters on a Marketing Cloud Account Engagement (Pardot) Form Textarea
Recent Articles
How to Limit Characters on a Marketing Cloud Account Engagement (Pardot) Form Textarea
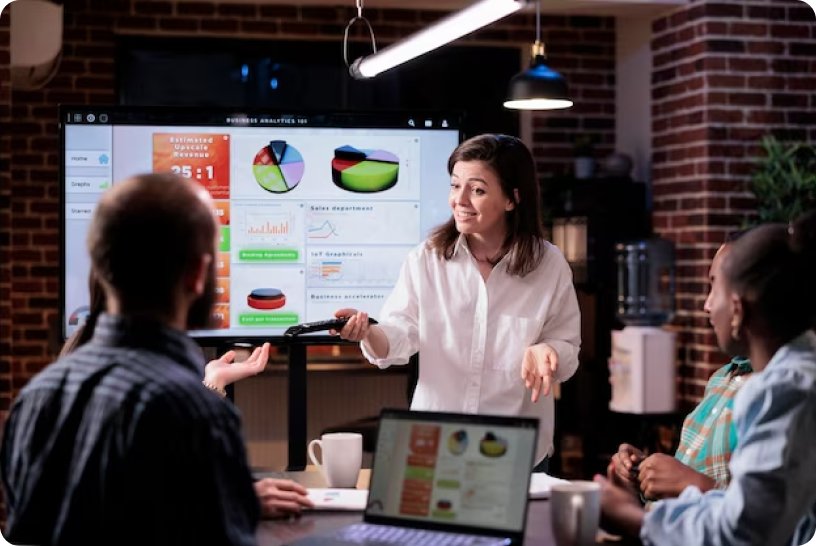
Recently we had a client request to limit characters on the comments textarea of a Marketing Cloud Account Engagement (Pardot) form. After searching high and low, we did find one beneficial blog on the subject, but it did not suit our purposes exactly, so we used that to build on and created our own. You can find it here written by Allie Cappetteli : https://thespotforpardot.com/2019/10/09/adding-character-limits-to-pardot-form-fields/
Let’s get started then!
The Code
Below you will find the code for this solution. The character limit is set to 10 (on line 8), which you can change to whatever you care to. Our use case called for 1000 characters.
<script type="text/javascript" src="https://ajax.googleapis.com/ajax/libs/jquery/3.4.1/jquery.min.js"></script>
<script>
$(document).ready(function() {
window.pardot.$("p.comments textarea").after("</p>
<p>
</p>
<p>
</p>
<p>
</p>
<p>
</p>
<p>
</p>
<p>
</p>
<p>
</p>
<p>
</p>
<p>
</p>
<p>
</p>
<p>
</p>
<p>
</p>
<p>
</p>
<p>
</p>
<p>
</p>
<p id='limit-text' style='color: #000000; font-weight: normal;'>Under character limit.</p>
<p>
</p>
<p>
</p>
<p>
</p>
<p>
</p>
<p>
</p>
<p>
</p>
<p>
</p>
<p>
</p>
<p>
</p>
<p>
</p>
<p>
</p>
<p>
</p>
<p>
</p>
<p>
</p>
<p>
</p>
<p>");
$('.comments textarea').on('keyup', function() {
var charMax = 10;
var charCount = $(this).val().replace(/s/g, '').length;
var charCountOver = charCount - charMax;
var charUnderLimit = "Under character limit";
var charOverLimit = charCountOver + " characters over";
if (charCount <= charMax) {
window.pardot.$("p.comments").removeClass("error");
window.pardot.$(".limit-text").remove();
window.pardot.$("p.submit input").prop('disabled', false);
} else {
window.pardot.$("p.comments").addClass("error");
window.pardot.$("p.submit input").prop('disabled', true);
}
if (charCountOver < 1) {
window.pardot.$("#limit-text").text(charUnderLimit);
} else {
window.pardot.$("#limit-text").text(charOverLimit);
}
});
});
</script>
Add the code to your Marketing Cloud Account Engagement (Pardot) form
- Open the form editor
- Navigate to the ‘Look and Feel’ editor page
- Select the ‘Below Form’ section and click the ‘Source’ icon
- Copy the code from above and paste the code into the editor 4. Click “Confirm & Save”
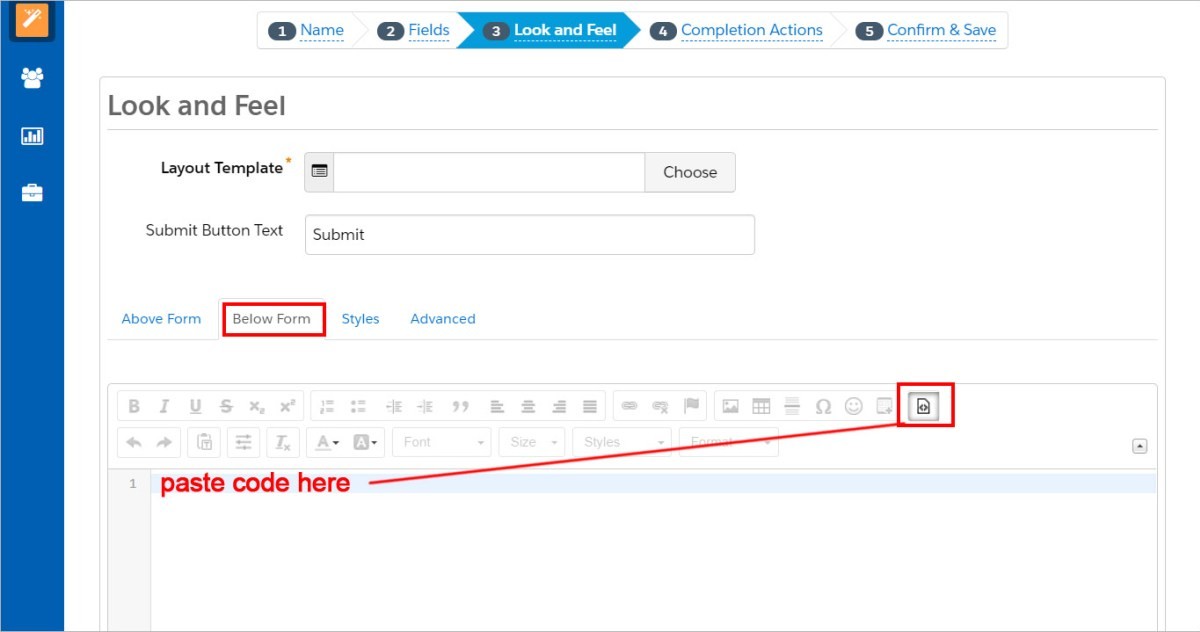
Add CSS class to the Comments textarea
- Navigate to the “Fields” page in the editor
- Click the edit button on the “Comments” form element. If you have not added the Comments’ textarea, do so now.
- Click the “Advanced” tab and enter “comments” in the CSS Classes field
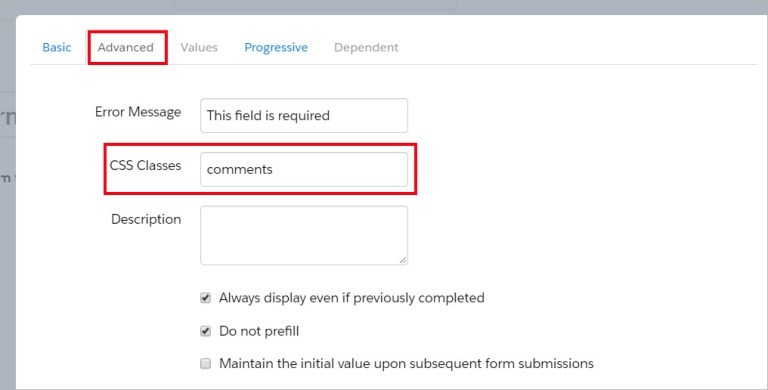
Click “Confirm & Save” and then test your form. That’s it! Good Job!
Other Settings in the Code
Change the Text Shown On Load
When the page opens up, there is text that shows under the comment field on load. Depending on if you elect to change some of the other criteria in the script, you may decide that you want to present different text to your site visitors when the form loads. In this example, you can update the red portion of the below code snippet on line 4 to update your text that is shown on load:
window.pardot.$("p.fieldName fieldType").after("0/100");
Change the Text to Show Different Text
If you want to change the text that appears at the bottom of your Comments field, simply change lines 13 and 14 between the quotes. var charUnderLimit = “Under character limit“; var charOverLimit = charCountOver + “characters over“;
Change the Count Text to Show Amounts Instead of Under/Over Limit
If you want to change the text at the bottom of your comments field to show the number of typed characters over the number of available characters, make these below changes:
- Remove Lines 13 and 14 and replace with a new limit
- Add a new Limit
- Update Line 25 and 27 with the new output
var charLimit = charCount+" / "+ charMax;
window.pardot.$("#limit-text").text(charLimit);
window.pardot.$("#limit-text").text(charLimit);
Adapting this Code for Different Field Types
One important thing to note here is that yes, this code can be just cut and pasted to run on any textarea field type in Marketing Cloud Account Engagement (Pardot), there are other field types that you will likely want to use this on. One of the most likely scenarios is a text field instead of a textarea. In this case, you must update the code to execute against this different field type. Please update the textarea section in lines 2 and 6 to say text.